1、FTP文件服务器的搭建:
软件下载:ftpserver;
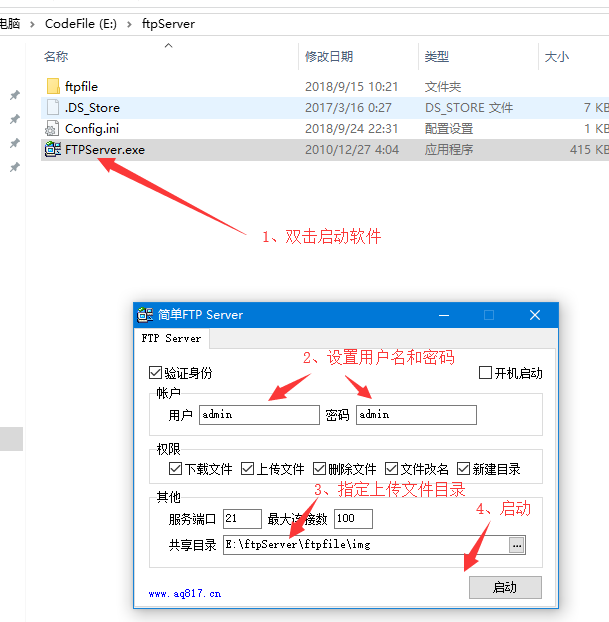
image.png
浏览器访问: ftp://127.0.0.1/
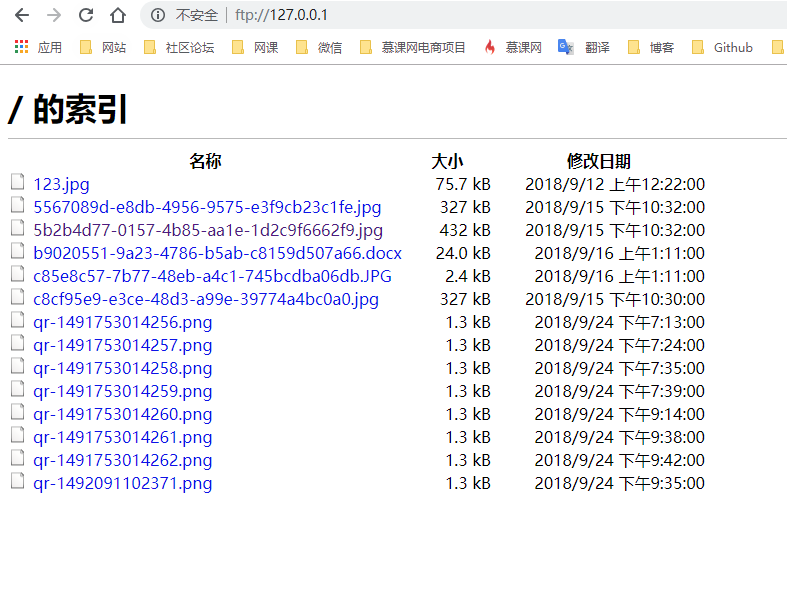
image.png
点击任意一个文件,就可以看到我们图片啦,前提是前面指定的目录里面有图片文件~
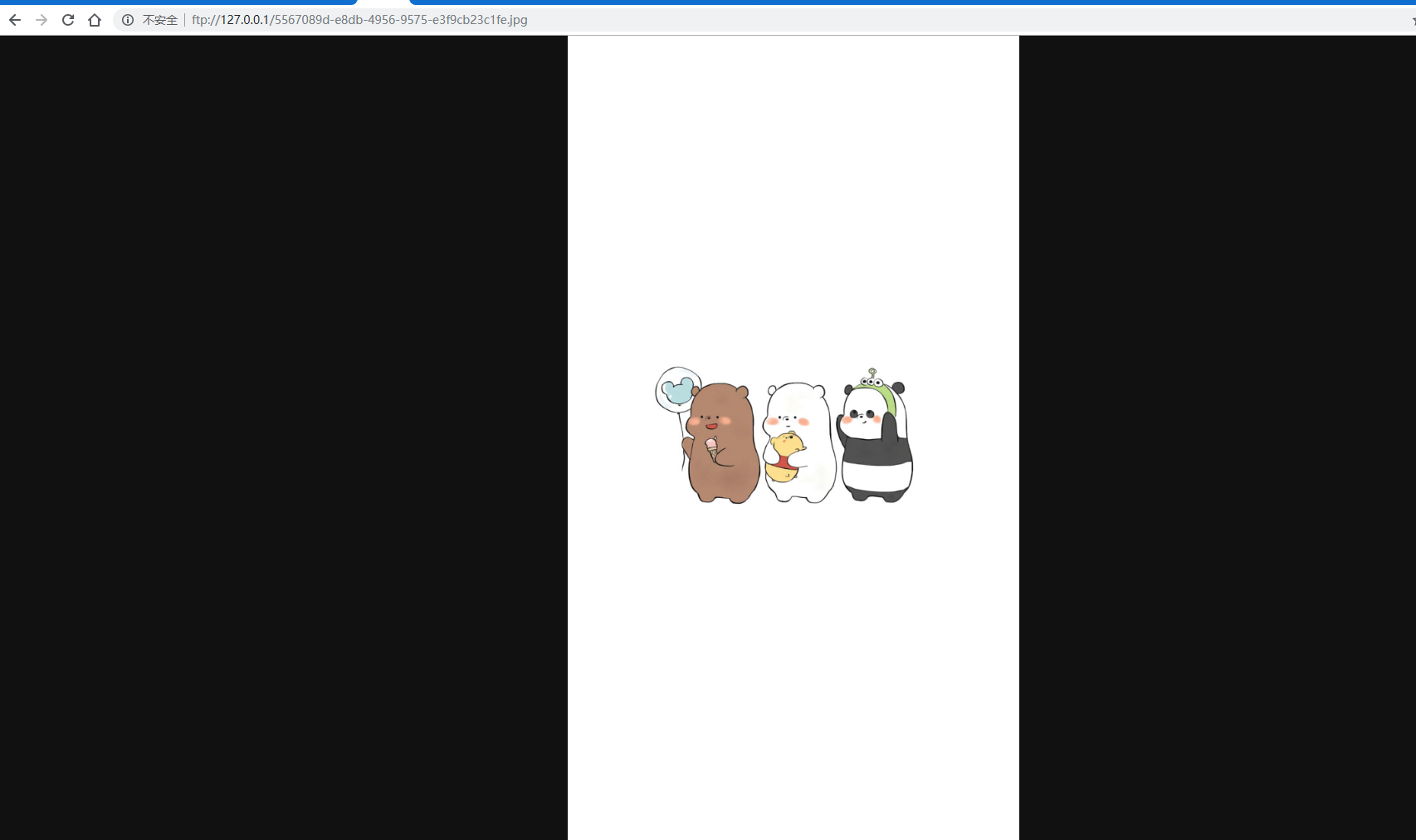
image.png
2、接口编写:
1、springmvc方法上传文件:
在ProductManageController
:中编写下面方法:*Controller
:
//springmvc文件上传接口
@RequestMapping("upload.do")
@ResponseBody
public ServerResponse upload(HttpSession session, @RequestParam(value = "upload_file",required = false) MultipartFile file, HttpServletRequest request){
User user=(User) session.getAttribute(Const.CURRENT_USER);
if(user==null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.NEED_LOGIN.getCode(),"未登录,请先登录");
}
if(iUserService.checkAdminRole(user).isSuccess()){
String path=request.getSession().getServletContext().getRealPath("upload");
String targetFileName=iFileService.upload(file,path);
String url= PropertiesUtil.getProperty("ftp.server.http.prefix")+targetFileName;
Map fileMap= Maps.newHashMap();
fileMap.put("uri",targetFileName);
fileMap.put("url",url);
return ServerResponse.createBySuccess(fileMap);
}else {
return ServerResponse.createByErrorMessage("当前登录者不是管理员,无权限操作");
}
}
这行代码需要注意的是@RequestParam(value = "upload_file",required = false) MultipartFile file
参数的传入,对应的是相关文件类属性。
public ServerResponse upload(HttpSession session, @RequestParam(value = "upload_file",required = false) MultipartFile file, HttpServletRequest request)
*Service
:
//文件上传方法实现
String upload(MultipartFile file, String path);
*ServiceImpl
:
//文件上传方法实现
public String upload(MultipartFile file,String path){
String fileName=file.getOriginalFilename();
//扩展名
//abc.jpg 我们要拿到jpg
String fileExtensionName=fileName.substring(fileName.lastIndexOf(".")+1);
//防止文件被覆盖,我们使用UUID生产的字符串作为文件名,这样用户上传同名的文件就不会被覆盖了
String uploadFileName= UUID.randomUUID().toString()+"."+fileExtensionName;
logger.info("开始上传文件...上传文件的文件名:{},上传的路径:{},新文件名:{}",fileName,path,uploadFileName);
//创建文件夹
File fileDir=new File(path);
if(!fileDir.exists()){
fileDir.setWritable(true);
fileDir.mkdirs();
}
//上传文件
File targetFile=new File(path,uploadFileName);
try {
file.transferTo(targetFile);
//文件上传成功
//将targetFile上传到我们的文件服务器
FTPUtil.uploadFile(Lists.newArrayList(targetFile));
//文件已经上传到FTP服务器上
//上传文件到文件服务器之后,删除我们Tomcat里面的文件,防止存储文件过多
targetFile.delete();
} catch (IOException e) {
logger.error("上传文件异常",e);
return null;
}
return targetFile.getName();
}
由于是直接讲文件上传到文件服务器,所以不涉及到数据库的操作~
2、富文本上传:
富文本我们选择的是simditor
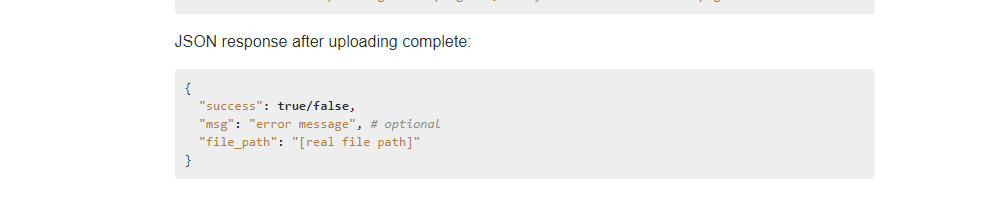
image.png
相关文档位置:
https://simditor.tower.im/docs/doc-config.html#anchor-defaultImage
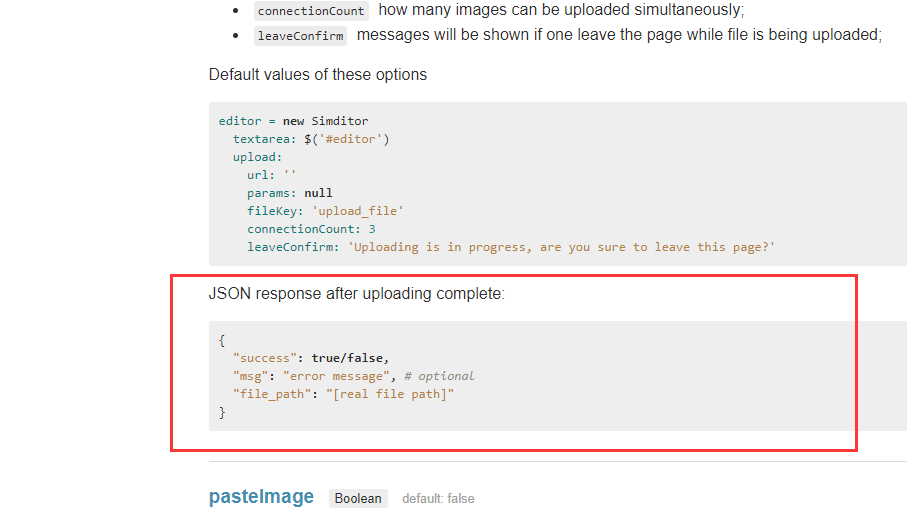
image.png
//富文本上传接口
@RequestMapping("richtext_img_upload.do")
@ResponseBody
public Map richtextImgUpload(HttpSession session, @RequestParam(value = "upload_file",required = false) MultipartFile file, HttpServletRequest request, HttpServletResponse response){
Map resultMap=Maps.newHashMap();
User user=(User) session.getAttribute(Const.CURRENT_USER);
if(user==null){
resultMap.put("success",false);
resultMap.put("msg","未登录,请先登录");
return resultMap;
}
//富文本中对于返回值有自己的要求,我们使用是simditor 所以要按照simditor的要求进行返回
if(iUserService.checkAdminRole(user).isSuccess()){
String path=request.getSession().getServletContext().getRealPath("upload");
String targetFileName=iFileService.upload(file,path);
if(StringUtils.isBlank( targetFileName)){
resultMap.put("success",false);
resultMap.put("msg","上传失败");
return resultMap;
}
String url= PropertiesUtil.getProperty("ftp.server.http.prefix")+targetFileName;
resultMap.put("success",true);
resultMap.put("msg","上传成功");
resultMap.put("ile_path",url);
response.addHeader("Access-Control-Allow-Headers","X-File-Name");
return resultMap;
}else {
resultMap.put("success",false);
resultMap.put("msg","当前登录者不是管理员,无权限操作");
return resultMap;
}
}
至于上传的upload
方法我们还是使用springmvc
中使用的方法~
3、测试接口:
接下来就是编写一个页面测试这两个方法啦
在index.jsp
页面中编写下面代码:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<html>
<body>
<h2>Hello World!</h2>
springmvc上传文件
<form name="form1" action="/manage/product/upload.do" method="post" enctype="multipart/form-data">
<input type="file" name="upload_file">
<input type="submit" value="springmvc上传文件">
</form>
富文本图片上传
<form name="form1" action="/manage/product/richtext_img_upload.do" method="post" enctype="multipart/form-data">
<input type="file" name="upload_file">
<input type="submit" value="富文本上传文件">
</form>
</body>
</html>
1、springmvc测试:
1

image.png
2
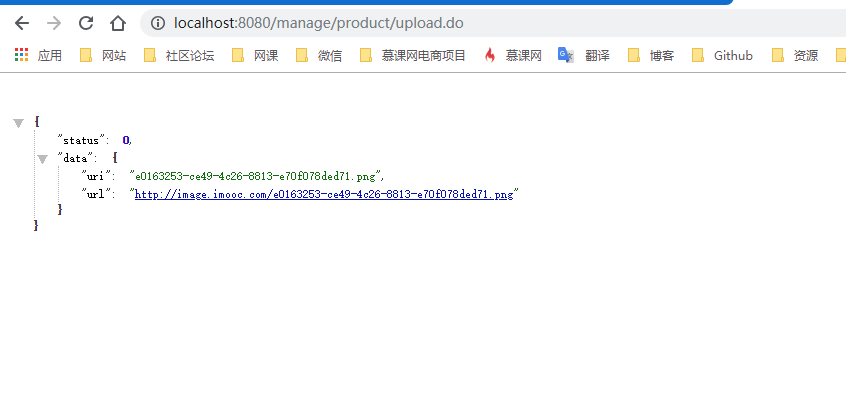
3
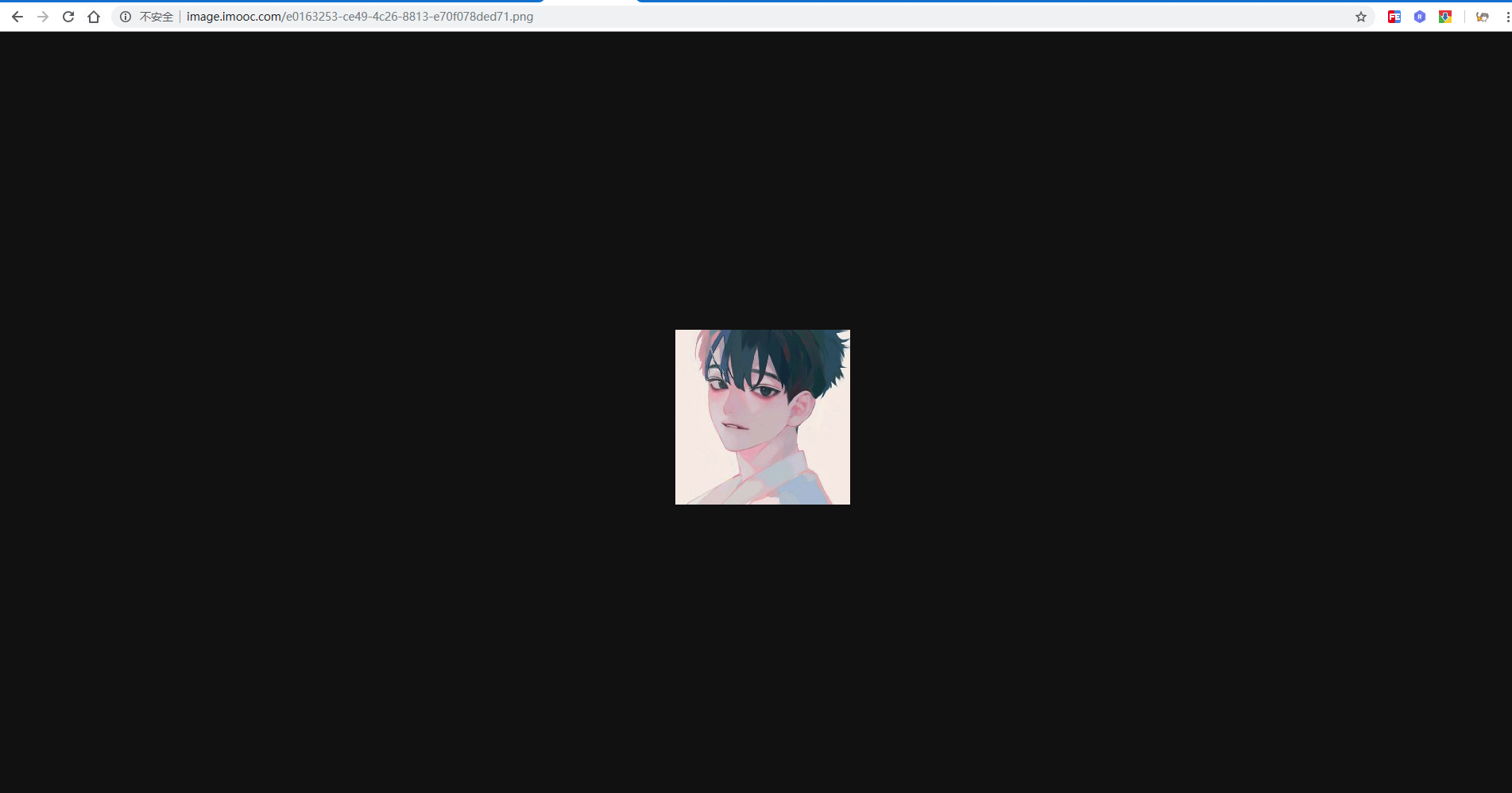
image.png
2、符文本测试:
1
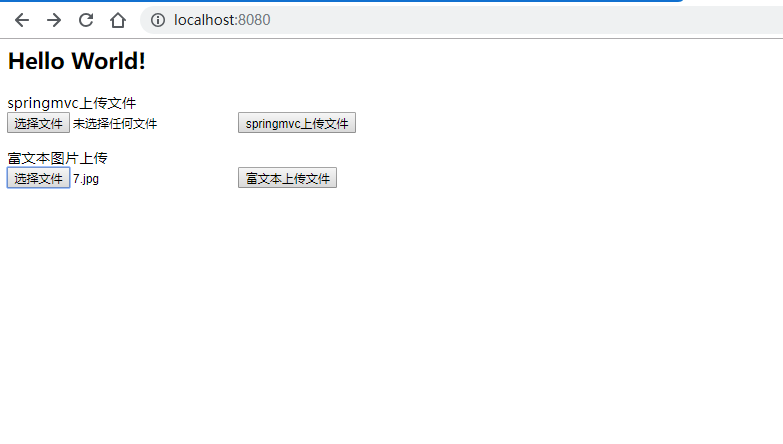
image.png
2
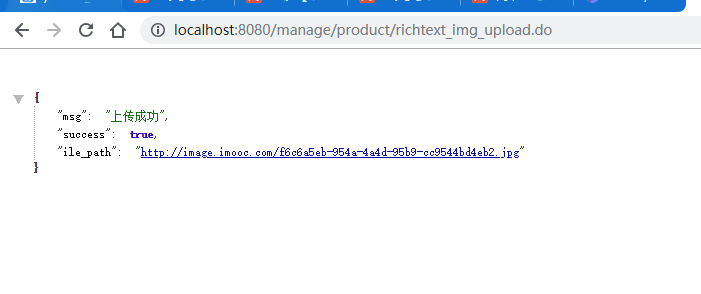
3
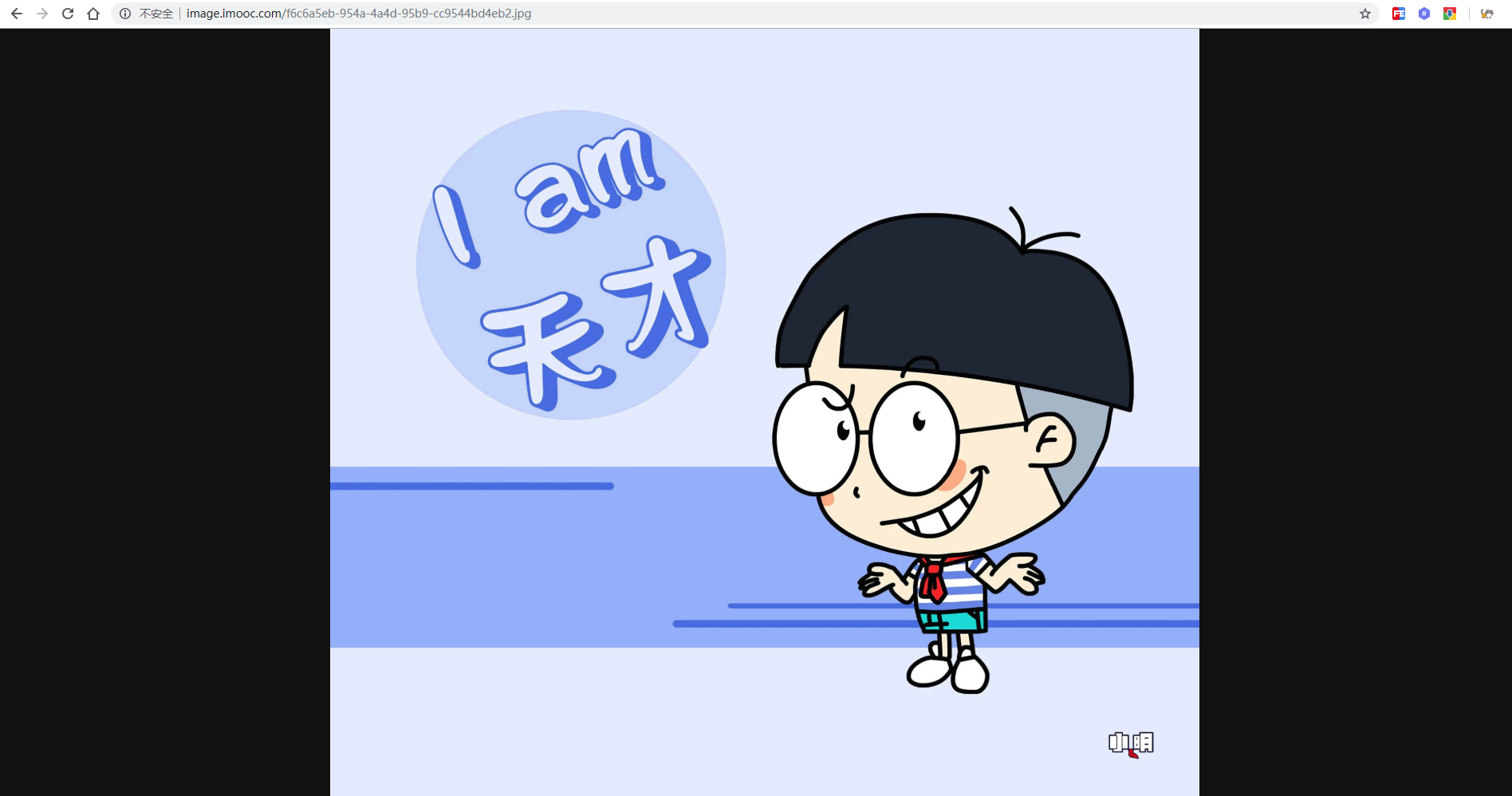
image.png